Get Authenticated and make your first API Call
Get authenticated using your Application Credentials
Firstly, you should get your application credentials (application-id, application-secret) from the Routee Platform.
Visit https://go.routee.net to Get Started with a free Account!
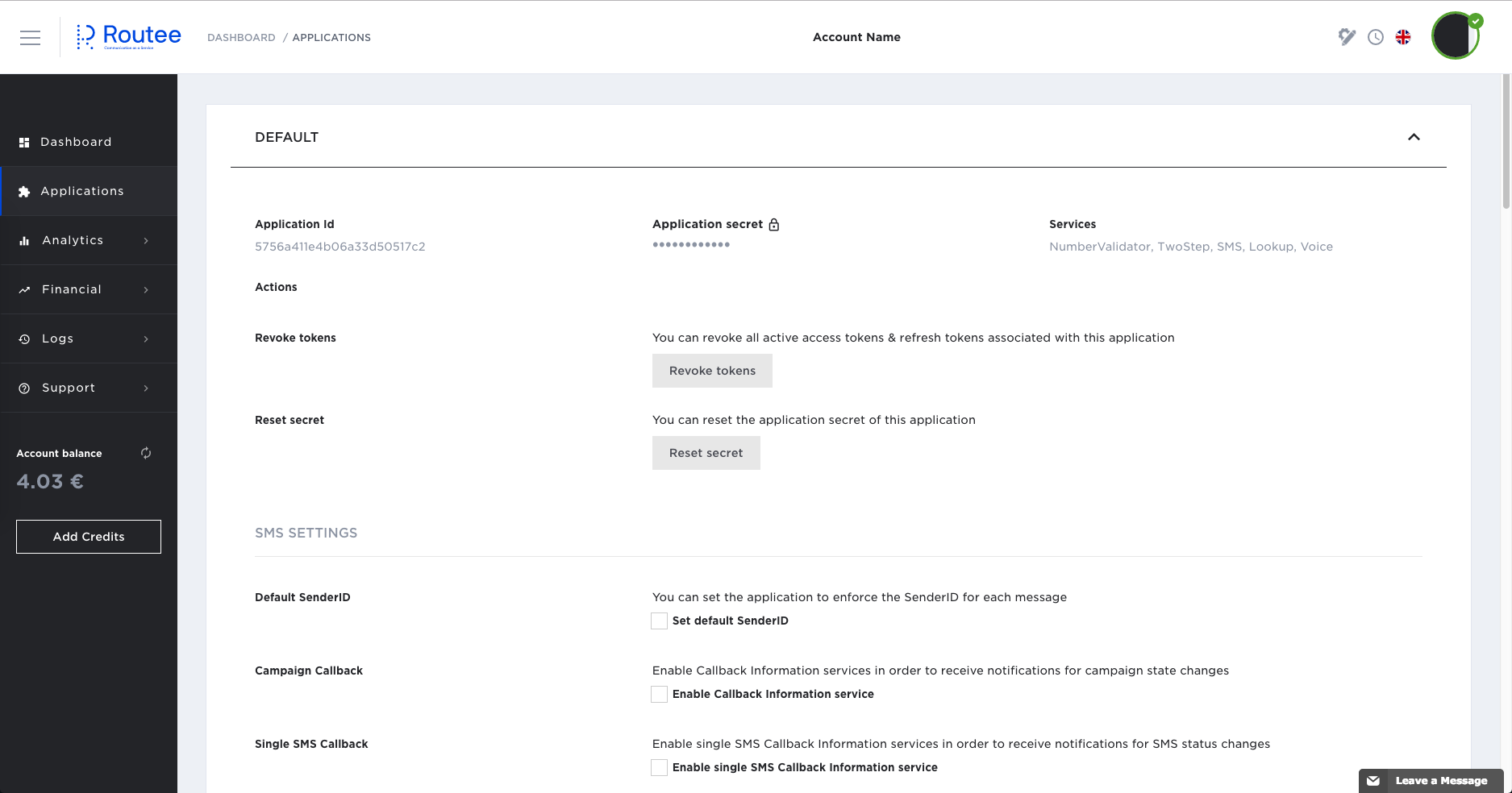
Applications Menu
Click on the lock icon to get the application secret (user password is required).
Then you should encode the applicationid:applicationsecret string to a Base64 string.
Having this string you are now able to exchange your application credentials for an access token by calling the Auth resource
The Authorization header of the request must include the word "Basic" followed by the Base64 encoded string. Each application is associated with an account. For more information about applications check here
Note. You can create multiple applications with different id and secret if that suits your integration case. Read more...
Example#
Application id | Application secret |
---|---|
5756a411e4b06a33d50517c7 | vb6QpjCIOG |
String before the encoding |
---|
5756a411e4b06a33d50517c7:vb6QpjCIOG |
Encode the resulting string using Base64
Base64 encoded string |
---|
NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c= |
Now you can exchange this string with an access token.
Request Headers
KEY | VALUE |
---|---|
Authorization | Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c= |
Content-Type | application/x-www-form-urlencoded |
Query Parameters
KEY | OPTIONAL | DESCRIPTION |
---|---|---|
grant_type | No | It must always have the value: client_credentials |
scope | Yes | The body can also contain a scope parameter in order to limit the permissions of the access token. For example, if an application sends only SMS it can request only the SMS scope. By default, if the scope parameter is omitted, then the token receives all the allowed scopes of the application |
curl --request POST \
--url https://auth.routee.net/oauth/token \
--header 'authorization: Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=' \
--header 'content-type: application/x-www-form-urlencoded' \
--data grant_type=client_credentials
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "grant_type=client_credentials");
Request request = new Request.Builder()
.url("https://auth.routee.net/oauth/token")
.post(body)
.addHeader("authorization", "Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=")
.addHeader("content-type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
var client = new RestClient("https://auth.routee.net/oauth/token");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddHeader("authorization", "Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=");
request.AddParameter("application/x-www-form-urlencoded", "grant_type=client_credentials", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://auth.routee.net/oauth/token",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "grant_type=client_credentials",
CURLOPT_HTTPHEADER => array(
"authorization: Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=",
"content-type: application/x-www-form-urlencoded"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
import http.client
conn = http.client.HTTPSConnection("auth.routee.net")
payload = "grant_type=client_credentials"
headers = {
'authorization': "Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=",
'content-type': "application/x-www-form-urlencoded"
}
conn.request("POST", "/oauth/token", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
require 'uri'
require 'net/http'
url = URI("https://auth.routee.net/oauth/token")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["authorization"] = 'Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c='
request["content-type"] = 'application/x-www-form-urlencoded'
request.body = "grant_type=client_credentials"
response = http.request(request)
puts response.read_body
var settings = {
"async": true,
"crossDomain": true,
"url": "https://auth.routee.net/oauth/token",
"method": "POST",
"headers": {
"authorization": "Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=",
"content-type": "application/x-www-form-urlencoded"
},
"data": {
"grant_type": "client_credentials"
}
}
$.ajax(settings).done(function (response) {
console.log(response);
});
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"authorization": @"Basic NTc1NmE0MTFlNGIwNmEzM2Q1MDUxN2M3OnZiNlFwakNJT0c=",
@"content-type": @"application/x-www-form-urlencoded" };
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"grant_type=client_credentials" dataUsingEncoding:NSUTF8StringEncoding]];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://auth.routee.net/oauth/token"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
[request setHTTPBody:postData];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
HTTP Response
{
"access_token":"string",
"token_type":"string",
"expires_in":"number",
"scope":"string,string,string",
"permissions":[
"string"
]
}
Response Parameters
KEY | DESCRIPTION |
---|---|
access_token | The generated access_token. This must be used in all requests. |
expires_in | Time in seconds that the token will expire. The token is set by default to expire in 1 hour (3600 seconds), or a new application can be created with the following options: 1 day (86400), 20 years (631139040) |
scope | The requested scopes. |
permissions | The permissions granted to the authenticated application. |
That's it! Now you can access all Routee services using the access_token of your application to the header of each resource!
Note. All requests made for a Routee Application are associated to the Routee Account. You can use Routee Platform to generate custom reports for all your activity.
Important!
All tokens that are issued from Routee's authorization server are valid for 1 hour. This allows better security for your HTTP calls. That means that once you get an HTTP response with a status code of 401 or 403, from any Routee API resource, you should issue a new token and repeat your previous request with the new token.
To issue a new token, you follow the same procedure of exchanging your application credentials through the authorization server.
Non-expiring Token
If by any reason you want a non-expirable token then you should create a new application on https://go.routee.net/#/management/applications or https://dev.routee.net/#/management/applications and at the dropdown Token Expiration Settings select the option Unlimited. When you exchange the new application ID & Secret with the oAuth resource the receiving token will never expire.
Resource reference here
Updated about 1 year ago